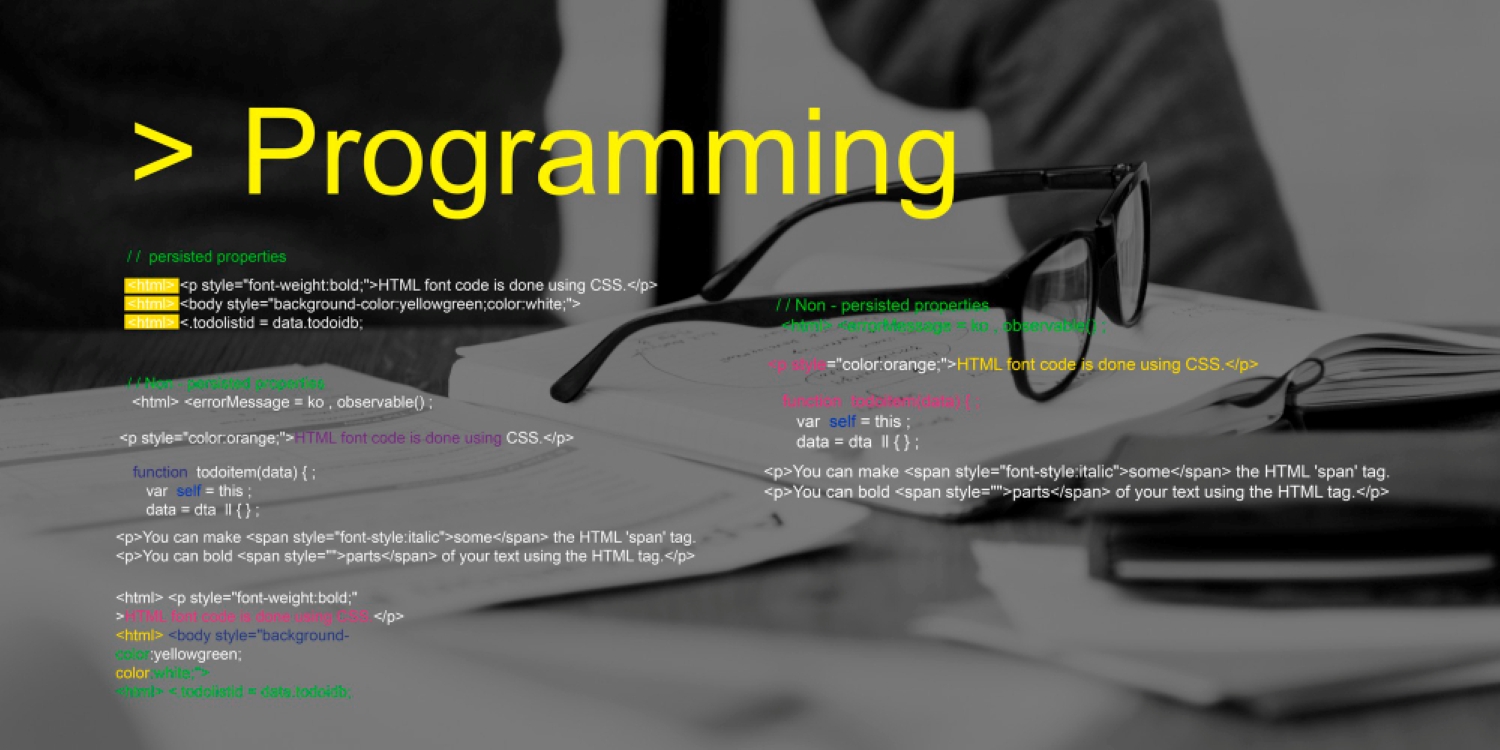
Unraveling the Mysteries: Mastering Logic in JavaScript and TypeScript
Learn how to construct robust applications by incorporating logical reasoning into your code. This journey will equip you with advanced problem-solving skills applicable to numerous programming contexts.
Understanding Logic: The Foundation of Programming
Before diving into JavaScript and TypeScript, let's establish a strong foundation in programming logic. This section will explore the fundamental principles that underpin all successful programs.
What is Logic in Programming?
In programming, logic refers to the set of rules and principles that govern how a program processes information and makes decisions. It's the backbone of any application, determining how data is manipulated, how conditions are evaluated, and ultimately, what actions the program takes. Think of it as the program's brain, enabling it to respond intelligently to various inputs and circumstances. Without logic, a program would simply be a collection of inert code, incapable of performing meaningful tasks.
Mastering logic is crucial because it directly impacts a program's correctness, efficiency, and maintainability. A well-structured, logically sound program is easier to understand, debug, and extend, significantly reducing development time and costs. Poor logic, on the other hand, can lead to bugs, unexpected behavior, and ultimately, a failed application.
AI Prompt: A colorful abstract representation of branching logic pathways, resembling a circuit board or a flowing river splitting into multiple streams.
Types of Logic: Declarative vs. Imperative
Programming logic can be broadly classified into two categories: declarative and imperative. Imperative programming focuses on *how* to achieve a result, specifying a step-by-step sequence of instructions. This is often seen in procedural languages like C or Pascal. In contrast, declarative programming focuses on *what* result is desired, leaving the *how* to the underlying system. This approach is prevalent in languages like Prolog or SQL. JavaScript and TypeScript, while predominantly imperative, incorporate elements of declarative programming through features like functional programming paradigms. Understanding these different approaches can help you choose the most effective way to solve a given problem. Learn more about enhancing your programming skills with ApnaGuru's comprehensive courses: https://aapn.in/M40X8YQX.
The choice between declarative and imperative approaches often depends on the specific problem and the programmer's preferences. For instance, if you're working with data transformations, a declarative approach might be more concise and easier to understand. Conversely, for complex algorithms with intricate control flow, an imperative approach might offer more fine-grained control.
Applying Logic to Problem Solving
Effective problem-solving in programming relies heavily on breaking down a problem into smaller, manageable parts, and then applying logical reasoning to each part. This involves identifying the inputs, outputs, and intermediate steps involved, and choosing the appropriate logical structures (like conditional statements, loops, and functions) to implement each step. This systematic approach helps prevent errors and makes the program easier to maintain.
Consider a program that calculates the area of a rectangle. First, you need to identify the inputs (length and width), the output (area), and the calculation (area = length * width). Then, you can use programming logic to structure the code, ensuring that the correct inputs are collected, the calculation is performed accurately, and the result is presented clearly. This seemingly straightforward example highlights the importance of carefully defining the problem's logic before even writing a single line of code. Expand your programming horizons with ApnaGuru; visit https://aapn.in/M40X8YQX to discover a wealth of learning resources.
Building Logic in JavaScript
JavaScript, a cornerstone of web development, provides a rich set of tools for building sophisticated logic into your applications. Let's explore some key features.
Control Flow Statements: if-else, switch
Control flow statements dictate the order in which instructions are executed. The if-else
statement allows a program to make decisions based on whether a condition is true or false. The switch
statement provides an alternative for handling multiple conditions more efficiently. These statements form the foundation for creating dynamic and interactive applications.
For example, consider a simple program that determines whether a number is positive, negative, or zero. You can use an if-else
statement to check the number's value and execute different code blocks depending on the outcome. Similarly, a switch
statement is handy when dealing with multiple potential values of a variable, such as checking the day of the week. The choice between if-else
and switch
is typically made based on readability and the number of conditions being checked. Master your JavaScript skills with ApnaGuru's specialized courses. Check out our offerings today at https://aapn.in/M40X8YQX.
Looping Constructs: for, while, do-while
Loops are essential for repeating code blocks multiple times. JavaScript provides several loop types, each suited for different situations. The for
loop is ideal for iterating over a known number of times, while the while
and do-while
loops repeat as long as a condition is true or false.
For instance, you might use a for
loop to iterate over the elements of an array, or a while
loop to process user input until a specific condition is met. Choosing the correct loop type significantly influences the code's clarity and efficiency. Understanding the differences and best practices for loop usage is critical for writing effective JavaScript applications. Enhance your coding abilities with ApnaGuru's practical courses; visit https://aapn.in/M40X8YQX to learn more.
Conditional Operators: &&, ||, !
Conditional (logical) operators combine multiple conditions to produce a single boolean result. The &&
(AND) operator returns true only if both operands are true, while the ||
(OR) operator returns true if at least one operand is true. The !
(NOT) operator inverts the truth value of an operand. These operators are crucial for expressing complex logical conditions within control flow statements and other parts of your code.
Consider a scenario where a user must be at least 18 years old *and* possess a valid driver's license to proceed. You would use the &&
operator to ensure both conditions are met. Conversely, if a user can proceed if they are either 18 or older *or* have parental consent, you would use the ||
operator. Mastering these operators allows for clear and concise expression of complex logic. Check out ApnaGuru's courses to level up your programming skills: https://aapn.in/M40X8YQX.
Logical Operators in JavaScript
JavaScript's logical operators, including AND, OR, and NOT, are fundamental to building complex decision-making processes in your programs. They enable you to construct conditions that involve multiple boolean expressions, allowing for sophisticated control flow.
For example, you might use logical operators to validate user input, where several conditions must be true before the input is deemed valid. Or, you might use them in game development to determine whether a player has met certain criteria to advance to the next level. Understanding and utilizing these operators effectively is a crucial skill for any JavaScript developer. To learn more, explore ApnaGuru's comprehensive curriculum: https://aapn.in/M40X8YQX.
Building Logic in TypeScript
TypeScript, a superset of JavaScript, adds static typing to enhance code maintainability and scalability. Let's see how type safety interacts with logic.
Type Safety and Logic
TypeScript's type system helps catch logical errors during compilation, preventing runtime surprises. By specifying data types, you constrain the values that variables can hold, reducing the likelihood of unexpected behavior due to incorrect data.
For instance, if your function expects a number, TypeScript will prevent you from accidentally passing a string, thereby avoiding potential runtime errors caused by incorrect data types. This increased type safety leads to more robust and predictable logic. Explore ApnaGuru's courses and strengthen your understanding of TypeScript; visit https://aapn.in/M40X8YQX.
Interfaces and Logic
Interfaces define the structure of objects in TypeScript, enabling you to enforce specific properties and their types. This ensures consistency in your data structures, improving the reliability of your logic.
If you're working with a function that operates on a particular object structure, an interface ensures that all objects passed to the function conform to the expected structure. This prevents unexpected behavior or errors during runtime. This approach enhances both the correctness and maintainability of your logic. Enhance your programming skills with ApnaGuru; check out our courses at https://aapn.in/M40X8YQX.
Generics and Logic
Generics allow you to write reusable code that can work with various types without compromising type safety. This improves code reusability and reduces redundancy.
Imagine writing a function to sort an array. Using generics, you can write a single sorting function that can handle arrays of numbers, strings, or custom objects, without compromising type safety. Generics thus promote both type safety and code reusability. Unlock your programming potential with ApnaGuru; visit https://aapn.in/M40X8YQX to discover our comprehensive range of courses.
Advanced TypeScript Concepts for Logic
TypeScript offers advanced features such as conditional types, mapped types, and utility types, that can enhance the expressiveness and safety of your code's logic. These features allow for more sophisticated type manipulations, leading to improved code clarity and reliability.
Conditional types allow you to define types based on certain conditions, allowing for complex type-level logic. Mapped types provide ways to transform existing types into new ones. Utility types offer pre-built type transformations to simplify development. These concepts enable more advanced logic within your TypeScript code, ensuring better type safety and efficiency. Explore the power of advanced TypeScript with ApnaGuru: https://aapn.in/M40X8YQX.
Practical Examples of Logic in JavaScript and TypeScript
Let's illustrate logical concepts with practical examples.
Example 1: Validating User Input
Validating user input is a common task involving several logical checks. In JavaScript, you might use conditional statements and regular expressions to ensure that the input matches the expected format, is within the acceptable range, and contains no malicious content. TypeScript adds an extra layer of safety by ensuring type consistency.
For example, if you have a form that collects a user's email address, you can use regular expressions to validate that the input conforms to a valid email format. In TypeScript, you can specify the expected type of the input field to further enforce data integrity. This combination of validation techniques enhances security and data integrity in your applications. To delve deeper into these topics, explore ApnaGuru's curriculum at https://aapn.in/M40X8YQX.
Example 2: Implementing a Search Algorithm
Search algorithms, like linear or binary search, involve substantial logic. In JavaScript, you'd use loops and conditional statements to compare values and determine whether a target element is present. TypeScript can enhance this by adding type checking to your search function's inputs and outputs.
Consider a program that searches for a specific item in a large dataset. You might implement a binary search, which requires logical operations to determine the middle element and compare it with the target. Using TypeScript, you can specify the type of the data being searched and the type of the expected result, increasing code readability and maintainability. For more advanced programming concepts, enroll in ApnaGuru's courses: https://aapn.in/M40X8YQX.
Example 3: Building a Simple Game
Game development involves implementing complex game logic. JavaScript is often used to handle game events, manage player actions, and update game states. TypeScript provides type safety to ensure the consistency of game data and prevent unexpected behavior.
For instance, you could use a game loop to update the game state and check for player interactions. TypeScript ensures that the game state and player data maintain type integrity throughout the game's lifecycle. Learn more about game development and other programming concepts with ApnaGuru's courses: https://aapn.in/M40X8YQX.
Advanced Techniques and Best Practices
As you progress, consider these advanced techniques to enhance your logic.
Refactoring for Clarity and Efficiency
Refactoring is the process of restructuring existing code without changing its external behavior to improve readability, maintainability, and efficiency. It is crucial for managing the complexity of large applications.
For instance, you might break down large functions into smaller, more focused ones, improving code clarity. You could also use design patterns or apply SOLID principles to improve modularity and maintainability. This makes it easier to understand, modify, and extend your code over time. ApnaGuru provides various courses that will aid in your programming journey. https://aapn.in/M40X8YQX
Debugging and Testing Your Logic
Debugging is the process of identifying and fixing errors in your code. Effective debugging requires careful consideration of your logic. You should employ debugging tools, such as breakpoints, logging, and inspection to trace the flow of execution and identify where errors occur. Thorough testing is essential to ensure that your logic functions correctly under various conditions.
Use systematic approaches to debugging, such as the divide-and-conquer strategy, to pinpoint the source of errors effectively. Comprehensive testing, including unit tests and integration tests, helps prevent regressions as you add new features and functionalities to your code. Consider ApnaGuru's courses to further your understanding of debugging and software testing. https://aapn.in/M40X8YQX.
Utilizing Libraries and Frameworks
Libraries and frameworks provide pre-built modules that can streamline development and reduce the amount of code you need to write. Many libraries and frameworks provide helpful utilities for common tasks, like data handling, networking, and user interface management.
Using these tools, you can often implement complex logic more efficiently. They provide pre-tested and optimized functions that you can integrate into your code. This greatly simplifies the development process and helps to ensure that your applications perform well and are reliable. Expand your knowledge of libraries and frameworks with ApnaGuru: https://aapn.in/M40X8YQX.
Conclusion: Mastering Logic in JavaScript and TypeScript
Mastering logic is fundamental to successful programming in JavaScript and TypeScript. By understanding control flow, looping, conditional operators, and advanced TypeScript features, you can build robust, efficient, and maintainable applications. Continuous learning and practice are key to honing your skills and tackling more complex programming challenges. Remember to prioritize code clarity, thorough testing, and effective use of available tools to build high-quality software. ApnaGuru can assist you in your learning journey. Visit https://aapn.in/M40X8YQX to learn more.