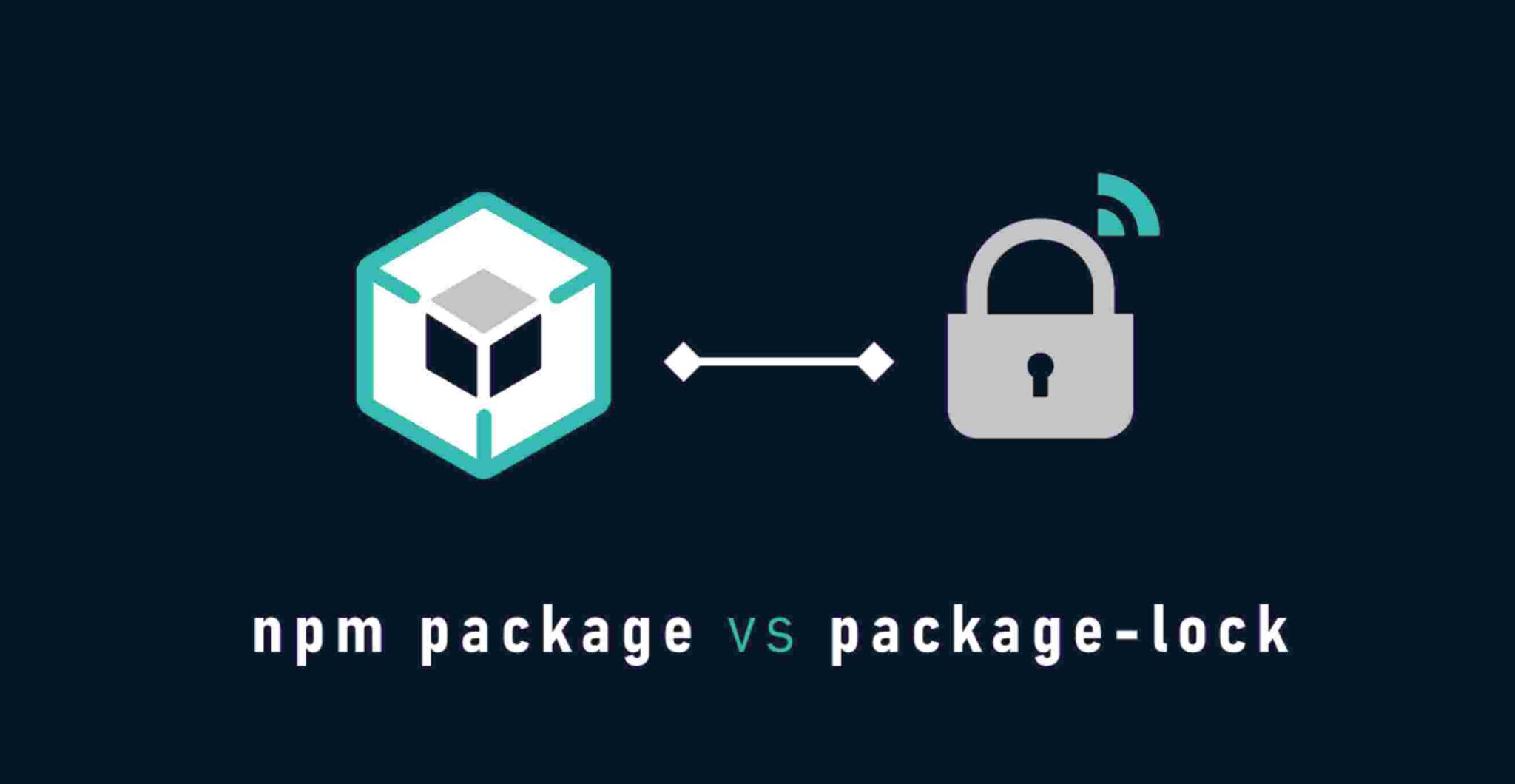
The Curious Case of package.json and package-lock.json: A Node.js Mystery Solved
Unraveling the Secrets of Node.js Dependency Management
package.json
and package-lock.json
? These seemingly simple files hold the key to dependency management, but their differences are often misunderstood. Prepare to delve into the intricacies of these vital components and unlock the secrets to a smoothly functioning project.The Enigma of package.json
and package-lock.json
: Unveiling the Differences
What is package.json
? The Heart of Your Node.js Project
Defining Project Metadata: Name, Version, and Description
The package.json
file serves as the foundational document for your Node.js project. It's essentially the project's identity card, containing vital metadata such as the project's name, version number, description, and author information. This information is crucial for others who might want to use or contribute to your project. Think of it as the project's resume, providing a concise summary of its key attributes.
For example, the name field clearly identifies the project, while the version helps track releases and updates. A descriptive description helps potential users understand the project's purpose. Well-structured metadata ensures clarity and facilitates easier project management. Maintaining accurate metadata in your package.json
is a cornerstone of good software development practice.
Specifying Dependencies: Production and Development
One of the primary roles of package.json
is to list the project's dependencies—external packages required for the project to function. It distinguishes between production dependencies (needed for the project to run in a production environment) and development dependencies (only needed during development, for tasks like testing or building). This distinction allows for streamlined deployments, as only essential packages are included in the production build.
Dependencies are declared using the dependencies
and devDependencies
fields. Each dependency is specified by its name and version range (e.g., "express": "^4.18.2"
). The version range allows for flexibility, ensuring the project continues to function even when minor updates to the dependencies are released. Precise version control, however, can be managed using the package-lock.json
file. Clear specification of dependencies ensures the project’s build remains consistent and reliable.
Managing Scripts: Automating Tasks
package.json
also provides a mechanism for defining scripts—commands that automate common development tasks. This allows developers to execute complex operations with simple commands, simplifying workflows and reducing errors. For instance, you might define a script for running tests, building the project, or starting a development server.
Scripts are defined within the scripts
field using key-value pairs where the key represents the command name (e.g., "start"
, "test"
, "build"
) and the value is the shell command to execute. This is a powerful feature that streamlines development and facilitates collaboration among team members by providing consistent and easily repeatable build procedures. The use of scripts promotes efficiency and prevents inconsistencies in development processes.
Understanding package-lock.json
: A Snapshot in Time
The Role of package-lock.json
in Dependency Management
Unlike package.json
, which specifies the *required* dependencies, package-lock.json
captures the *actual* dependencies installed in your project. It's an automatically generated file that meticulously records every dependency, including their exact versions and their dependencies' versions (and so on, forming a dependency tree). This provides a complete, reproducible record of the project's dependency structure.
This level of detail is critical for ensuring consistency across different environments and developers. It avoids the issues of unexpected behavior arising from different versions of dependencies. This file ensures that everyone working on the project will have the exact same dependencies, reducing the possibility of errors related to incompatible versions.
Ensuring Reproducibility: Locking Down Versions
The primary benefit of package-lock.json
is its ability to lock down dependency versions. Once generated, it ensures that the exact same dependencies are installed every time, regardless of who installs or on what system they install it. This reproducibility is vital for large projects and in collaborative environments, where consistency is key.
Without package-lock.json
, there's a risk of different developers installing different versions of dependencies, leading to inconsistencies and potential build or runtime errors. This file serves as a safety net that prevents such issues. It’s crucial for creating reproducible builds and for facilitating seamless deployment in various environments.
Resolving Dependencies: A Deep Dive
package-lock.json
also plays a crucial role in dependency resolution. It contains a detailed tree of all the dependencies, their versions, and their relationships. This enables npm (or yarn) to efficiently resolve dependencies, handling complex scenarios where multiple packages depend on different versions of the same dependency. This complex resolution is handled automatically and transparently.
The resolution process ensures that the dependencies are installed in a compatible manner, avoiding version conflicts. This is particularly important when dealing with a large number of dependencies, where manual resolution would be extremely tedious and prone to errors. This automatic resolution is a significant advantage, ensuring efficient and error-free dependency management.
Key Differences: Purpose, Dependency Management, and Versioning
Purpose: A Tale of Two Files
package.json
defines the project's metadata and lists the *required* dependencies, whereas package-lock.json
records the *actual* dependency tree, including exact versions. package.json
is for human readability and defining the project's intention; package-lock.json
is for machine readability and ensures reproducible builds. One is a statement of intent, the other a record of fact.
Think of package.json
as the recipe, outlining the ingredients (dependencies) needed for the project. package-lock.json
is then the detailed shopping list, specifying the exact brands, quantities, and versions of each ingredient, ensuring consistency in the final outcome. They complement each other to ensure successful project execution.
Dependency Management: The Human Touch vs. the Machine
package.json
allows for flexible version ranges in specifying dependencies, giving developers control over which versions are acceptable. package-lock.json
fixes the exact versions, providing a snapshot of the dependency tree. This ensures that the build process is reproducible and consistent across different environments.
The human-driven aspect of package.json
offers flexibility and control, while the machine-driven aspect of package-lock.json
ensures reproducibility. They represent two sides of the same coin—human intent and machine execution—working together for robust dependency management. This balance is crucial for effective project management.
Versioning: Explicit vs. Implicit
package.json
uses semantic versioning (SemVer) to specify dependency versions, allowing for flexibility in minor or patch updates. package-lock.json
locks down the exact versions of all dependencies, removing ambiguity. This explicit versioning in package-lock.json
is key for ensuring consistency and avoiding version conflicts.
The implicit versioning in package.json
provides flexibility, allowing for updates within a defined range. The explicit versioning in package-lock.json
guarantees that the exact same versions will be used each time, eliminating any discrepancies. This controlled approach minimizes the risk of errors related to version mismatches.
When to Update Each File: Best Practices
Updating package.json
: Intentional Changes
You should only update package.json
when you intentionally add, remove, or modify a dependency. This is usually done when you decide to include a new library or upgrade an existing one. Any changes here should be well-considered, as they directly impact the project's functionality.
Before making any changes, carefully consider the implications of adding or upgrading dependencies. Review the documentation and ensure compatibility with the rest of your project. Always test thoroughly after any changes to ensure they do not introduce unintended consequences. Well-planned changes in package.json
maintain project stability and functionality.
Updating package-lock.json
: The Automatic Update
package-lock.json
is usually updated automatically by npm (or yarn) whenever you install, update, or remove a dependency. You should not manually edit this file unless you understand the potential consequences, as doing so can break your project's dependency tree. Leave this file to be managed automatically by the package manager.
Directly modifying package-lock.json
is generally discouraged, as it can lead to inconsistencies and conflicts. The automated update mechanism ensures integrity and consistency, minimizing the risk of errors. Allowing the package manager to handle updates guarantees a reliable and stable dependency structure.
The Crucial Interplay: A Symphony of Files
package.json
and package-lock.json
work in tandem to ensure seamless dependency management. package.json
provides the high-level specifications, while package-lock.json
provides the detailed implementation. They must work together for efficient and reliable dependency handling.
The interplay between these two files is vital for a robust and reliable project. package.json
provides the blueprint, while package-lock.json
provides the detailed execution plan, ensuring the project is built consistently and reliably. This synergy is crucial for successful software development.
The Golden Rule: Which File Should You Modify?
Modifying package.json
: The Developer's Directive
As a developer, you should focus on modifying package.json
. This is where you specify the dependencies your project requires. Changes to package.json
will trigger an update in package-lock.json
, ensuring the changes are reflected in the dependency tree.
This separation of concerns keeps things clean and prevents accidental corruption of the dependency tree. Concentrating on package.json
for dependency changes allows for clear tracking of intended modifications, promoting efficient project management. This focused approach simplifies development and maintenance.
Leaving package-lock.json
Alone: Unless You Know What You're Doing
Unless you have a very specific reason (like resolving conflicts after merging branches), avoid directly modifying package-lock.json
. The file is automatically managed by npm or yarn, and direct modification can lead to unexpected problems and break your project.
Manually altering this file can easily introduce inconsistencies and break the carefully constructed dependency tree. Always prefer using the package manager to manage dependencies and let it handle updating this file. This prevents accidental errors and ensures the integrity of your project's dependencies.
The Exception: Resolving Conflicts
One exception to this rule is when merging branches, especially in collaborative environments. You might encounter conflicts in package-lock.json
. In these cases, carefully examine the differences and merge them responsibly, ensuring the final version reflects the correct dependency structure.
Such conflicts require careful attention to detail and thorough understanding of the changes. Prioritize resolving these issues to avoid future problems. When merging, always test thoroughly to ensure the changes haven’t introduced any unforeseen issues into the project.
Conclusion: Harmony in Dependency Management
package.json
and package-lock.json
are essential components for managing dependencies in your Node.js projects. Understanding their differences and how they work together is crucial for ensuring project consistency, reproducibility, and maintainability. By following the guidelines outlined above, you'll create a more robust and reliable project.
Proper understanding and management of these two files are vital for efficient and error-free dependency management. Their interplay ensures that everyone working on a project has the same dependencies, irrespective of their individual systems or environments, reducing the risk of build inconsistencies and promoting collaboration.