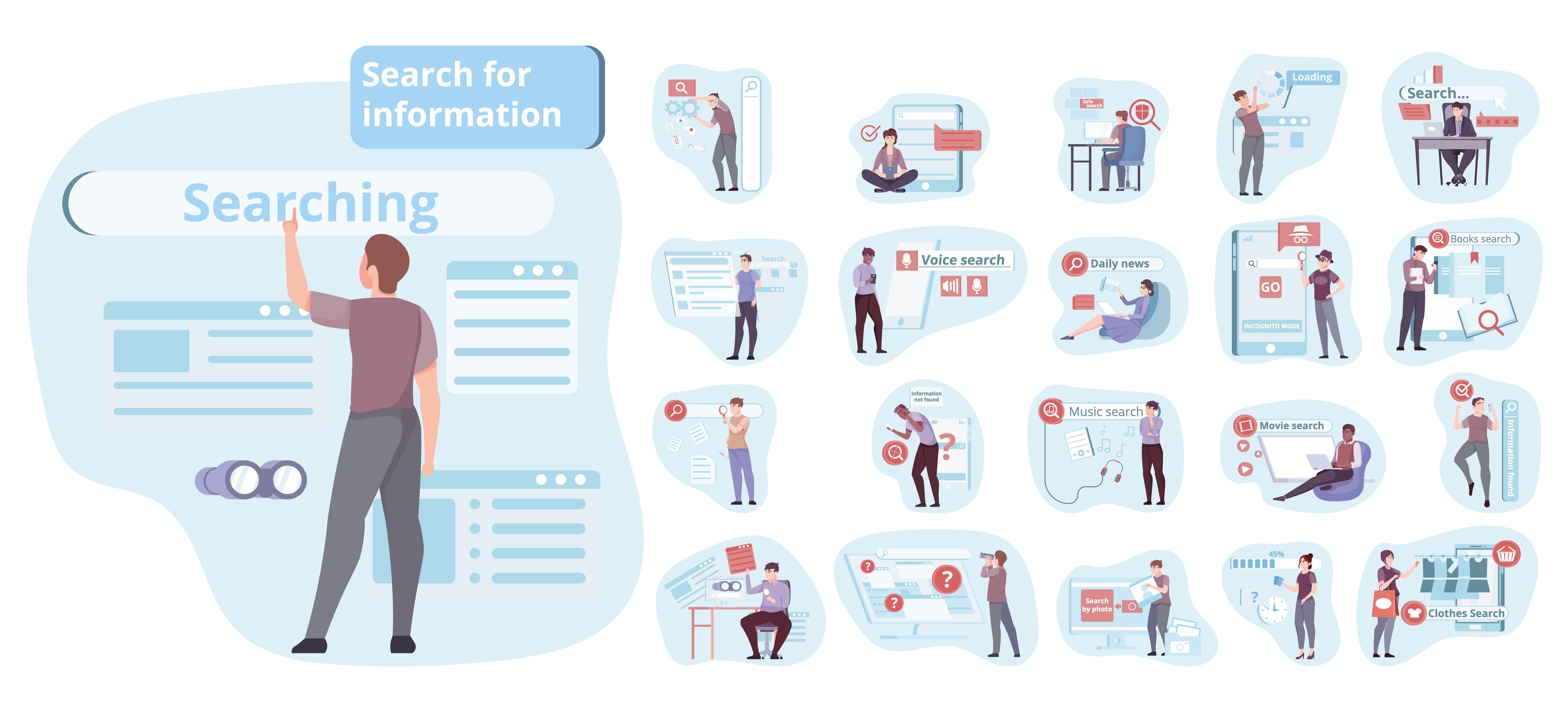
Unlocking Web Development Secrets: DOM Manipulation and Web Components
Introduction to DOM Manipulation and Web Components
What is DOM Manipulation?
The Document Object Model (DOM) is a programming interface for HTML and XML documents. DOM manipulation involves changing the structure, style, or content of an HTML document using JavaScript. It allows you to dynamically update your web page, making it interactive and responsive. For example, you could use DOM manipulation to change the text of a paragraph, add a new element to the page, or change the style of an element. Learning DOM manipulation is crucial for modern web development, laying the foundation for more advanced techniques.
Consider a scenario where a user clicks a button. Through DOM manipulation, you could then dynamically change the content on the page, perhaps displaying more information or hiding certain sections. This is how many interactive websites function. Without DOM manipulation, web pages would be static and far less engaging.
Understanding Web Components
Web components represent a significant advancement in web development. They are reusable custom HTML elements that encapsulate their own functionality, styling, and templates. Think of them as self-contained modules you can easily plug into your web pages, promoting code reusability, and simplifying maintenance. This means you can create a component once and then reuse it throughout your project or even across different projects without worrying about conflicts.Learn more about web components with ApnaGuru!
The beauty of web components is their modularity. If you need to update a specific component, you can do so without affecting other parts of your application. This is a massive improvement over monolithic JavaScript code, where a single change could inadvertently break other sections. Web components promote a cleaner, more maintainable codebase.
Check out ApnaGuru's courses on advanced web components.
Benefits and Advantages of Using Web Components
Encapsulation and Reusability
Web components excel at encapsulation – they bundle their HTML, CSS, and JavaScript into one neat package, preventing style conflicts and promoting clean code organization. This encapsulation enables easy reuse across projects, saving developers valuable time and effort. You create a button component once, and then use it multiple times across your site or even in different projects. No more copy-pasting code!
Imagine developing a complex application with many similar UI elements. With web components, you create each element once and reuse it throughout the app. This significantly reduces code duplication, making your codebase smaller and easier to maintain. The reusability aspect alone makes web components worth learning.Enroll in ApnaGuru's web development courses to master this!
Improved Performance
Web components often lead to performance improvements. The encapsulation and shadow DOM features help isolate components, reducing the risk of style conflicts and unintended side effects. This streamlined approach can result in faster load times and a smoother user experience. Browsers can optimize the rendering of web components more efficiently than handling scattered, unorganized code.
Because web components are self-contained, the browser can efficiently parse and render them. This improves overall page load time, crucial for user experience and SEO. Imagine your website loading faster – this directly improves user satisfaction and engagement.ApnaGuru can teach you how to build highly efficient web components!
Enhanced Maintainability
Maintaining a large codebase can be a nightmare. Web components tackle this by promoting modularity. If a bug is found in a specific component, you can fix it without affecting other parts of the application. This targeted approach makes debugging and updates much simpler and less error-prone. The compartmentalization reduces the ripple effects of changes.
Consider a scenario where you need to change the styling of a button in a large application. With web components, you only need to adjust the CSS within the button component itself. Without web components, you might need to hunt down and change the styling in multiple places, potentially introducing more bugs. This highlights the importance of maintainability.
Upgrade your skills with ApnaGuru's in-depth web component courses.
Better SEO
Web components contribute indirectly to improved SEO. Faster load times (due to performance improvements) positively impact SEO rankings. Search engines prefer fast-loading websites, and web components help achieve this. Furthermore, the structured and organized codebase can make it easier for search engine crawlers to index your content, leading to better visibility.
A faster website means better user experience, which is a critical ranking factor for search engines. By using web components to create a more efficient website, you are essentially boosting your site's SEO performance. The benefits extend beyond just the technical aspects of your code.ApnaGuru's courses will show you how to combine web components with SEO best practices.
Creating Web Components: A Step-by-Step Guide
Defining a Custom Element
Creating a web component begins by defining a custom element using the customElements.define()
method. This method takes two arguments: the element's name and a class that defines the component's behavior. The name should be in kebab-case (e.g., my-button
).
Let’s create a simple button:
class MyButton extends HTMLElement { constructor() { super(); this.attachShadow({ mode: 'open' }); this.shadowRoot.innerHTML = `<button>Click Me</button>`; }}customElements.define('my-button', MyButton);
This code defines a custom element named my-button
that renders a simple button. This is the basic structure for building more complex components. The key is understanding how to extend the HTMLElement
class.
Adding Styles and Functionality
Once you define your custom element, you can add styles using CSS and functionality using JavaScript. You can either inline the styles or use a separate stylesheet. The JavaScript functions will define how the component interacts with the user and the rest of the webpage.
We can improve our button by adding some styling within the shadow DOM:
class MyButton extends HTMLElement { constructor() { super(); this.attachShadow({ mode: 'open' }); this.shadowRoot.innerHTML = `<style>button { background-color: blue; color: white; }</style><button>Click Me</button>`; }}customElements.define('my-button', MyButton);
Here we added a basic CSS style to change the button's background color and text color. You can add more sophisticated styling and event handling to create complex behaviors. Learn more with ApnaGuru!
Using Shadow DOM
Shadow DOM is a crucial part of web components. It encapsulates the component's internal structure (HTML), styles (CSS), and behavior (JavaScript) from the rest of the page. This isolation prevents style conflicts and makes components reusable without worrying about overriding existing styles. The attachShadow()
method with mode: 'open'
allows access to the Shadow DOM for styling and event handling.
Shadow DOM is essential for maintaining the component’s independence. Imagine using a button component on a webpage with specific styles. Without Shadow DOM, the component's styles might conflict with the page's styles, leading to unpredictable results. Shadow DOM prevents this by keeping the component's styling completely isolated.
Expand your expertise with ApnaGuru’s comprehensive web component curriculum.
Example: A Simple Button Component
Let's create a more complete example of a custom button component that changes its text when clicked:
class MyButton extends HTMLElement { constructor() { super(); this.attachShadow({ mode: 'open' }); this.shadowRoot.innerHTML = `<style> button { background-color: #4CAF50; border: none; color: white; padding: 15px 32px; text-align: center; text-decoration: none; display: inline-block; font-size: 16px; } </style> <button>Click Me</button>`; this.shadowRoot.querySelector('button').addEventListener('click', () => { this.shadowRoot.querySelector('button').textContent = 'Clicked!'; }); }}customElements.define('my-button', MyButton);
This code demonstrates a button that changes its text upon clicking. It highlights how to incorporate event listeners and how to manipulate the component’s internal elements. This example builds upon the previous examples to show the power and flexibility of web components. Start your journey to mastering web components with ApnaGuru!
Advanced Web Component Techniques
Working with Attributes and Properties
Web components allow you to interact with attributes and properties. Attributes are strings you set on the HTML element, while properties are JavaScript values. You can use these to dynamically control the behavior and appearance of the components. Using attributes and properties allows for a more flexible and dynamic component design. The ability to pass data into a component makes them incredibly powerful.
Consider a button component with a text attribute. The value of this attribute can be used to set the text of the button itself. This means you don't have to hardcode the button text – you can control it dynamically from the outside. This level of control is a significant advantage of using properties and attributes.
Take your web development skills to the next level with ApnaGuru!
Handling Events
Handling events is essential for interactive components. You can add event listeners within your custom element to respond to user interactions like clicks, mouseovers, and keyboard inputs. This allows you to create components that react dynamically to user actions, making them interactive and engaging.
For example, in our button component, we added an event listener for the click event. This listener changes the button’s text when clicked. Event handling is critical for creating components that are more than just static elements. It’s what makes the components responsive and interactive. This responsiveness is crucial for a good user experience.
Learn how to create interactive web components with ApnaGuru.
Using Slots for Content Projection
Slots provide a powerful mechanism for injecting content into a web component from the outside. This allows you to customize the component’s behavior without modifying its internal structure. You can define slots within the component’s template and then use the slot element to insert external content into those designated areas.
Imagine a card component with slots for a title, image, and description. You could then use this card component multiple times, populating each instance with different content. This is very flexible, allowing for extensive customization without changing the component’s underlying code. Slots enable the creation of reusable and highly adaptable components.
Master the art of content projection with ApnaGuru’s advanced web component training.
Communication between Components
Communication between components is crucial for building complex applications. Several methods facilitate this, including custom events, properties, and attribute changes. Each method has strengths and weaknesses; choosing the right method depends on the specific communication needs.
Custom events are especially useful for unidirectional communication, where one component triggers an event that others can listen for. Properties and attribute changes work well for bidirectional communication. Understanding the different methods for component communication allows you to design more sophisticated and efficient applications.
ApnaGuru's comprehensive courses cover all these advanced techniques!
Real-World Applications of Web Components
Building Reusable UI Components
One primary application is building reusable UI components. Creating buttons, input fields, navigation menus, or even complex widgets as web components allows for consistency and easy maintenance across an entire website or application. This drastically reduces the amount of code and makes maintenance easier.
Imagine having to update the styling of a button used across a website with hundreds of pages. Without web components, this could be a time-consuming and error-prone task. But with web components, you only need to change the styling in one place – the button component itself. This simplifies the update process significantly.
Creating Custom Elements for Specific Applications
Web components are valuable for building custom elements specific to a particular application. This ensures seamless integration and maintainability, allowing developers to create specific components that perfectly match the application's requirements without conflicts or side effects.
Imagine developing a mapping application. You might create a custom component for displaying map markers or for handling user interactions with the map. These components would be custom-designed for your specific application. They will seamlessly integrate without worrying about conflicting styles or behaviors with other elements.
Explore ApnaGuru's expert-led courses and learn to craft specialized components!
Integrating with Existing Frameworks
Web components integrate well with various JavaScript frameworks (React, Angular, Vue). Their modular nature makes them compatible with existing frameworks without much friction, allowing developers to leverage the strengths of both web components and their preferred framework.
Using web components with existing frameworks can lead to improved code organization and maintainability. Web components handle the UI element, while the framework handles the overall application logic. This division of responsibilities leads to a cleaner architecture. This flexibility and compatibility make them valuable in a wide range of development scenarios.
Master web component integration with ApnaGuru’s specialized courses.
Conclusion
DOM manipulation and web components are powerful tools for modern web development. Understanding and utilizing these technologies allows developers to create efficient, reusable, and maintainable web applications. By mastering the concepts of encapsulation, shadow DOM, and component communication, you can unlock the full potential of these technologies. Start building efficient, modular web applications today!