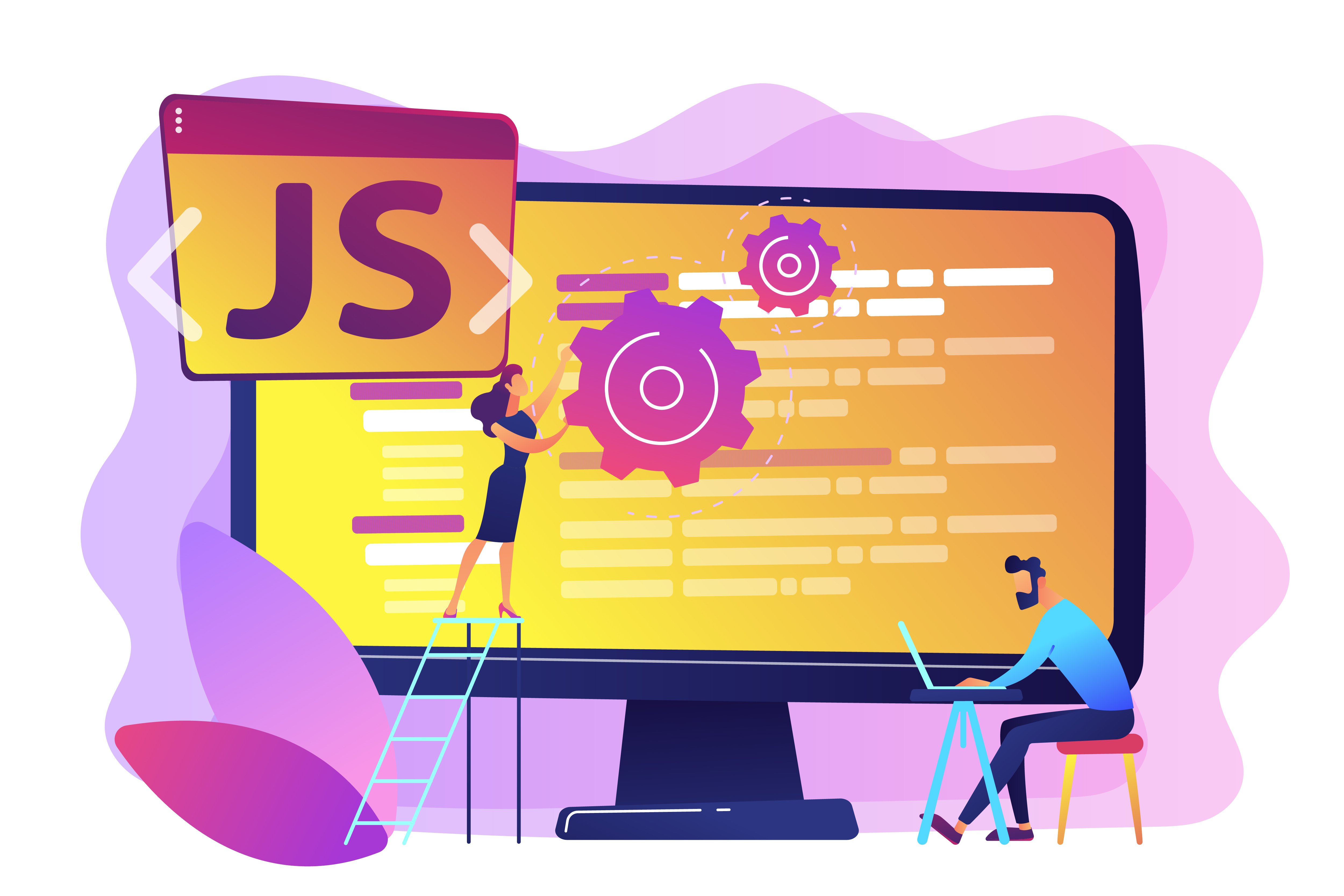
Unraveling the Mysteries: Mastering JavaScript Debugging, Troubleshooting, and Reverse Engineering
Introduction to JavaScript Debugging, Troubleshooting, and Reverse Engineering
Before we dive into the specifics, let's establish a clear understanding of the three core concepts: debugging, troubleshooting, and reverse engineering.
What is Debugging?
Debugging is the systematic process of identifying and removing errors from computer programs. It's like being a detective, meticulously tracing the steps of your code to find the culprit behind unexpected behavior. In JavaScript, this often involves using browser developer tools or IDE debuggers to step through your code line by line, inspecting variables, and identifying the source of problems. Learn more about advanced debugging techniques at ApnaGuru!
Imagine you're building a calculator. If it consistently returns wrong answers, debugging helps you pinpoint the flawed calculation, perhaps a misplaced operator or incorrect variable assignment. Debugging is crucial for ensuring software quality and reliability. Effective debugging skills are invaluable for any developer. Efficient debugging starts with understanding the flow of data through your code and its interactions with various parts of the program.
function add(a, b) {
return a - b; // Mistake: Subtraction instead of addition
}
console.log(add(5, 3)); // Expected 8, but returns 2
What is Troubleshooting?
Troubleshooting is a broader term than debugging. It involves identifying and resolving any problem, not just code errors. This could include anything from network connectivity issues to configuration problems or even hardware malfunctions. While debugging focuses on code, troubleshooting encompasses a wider range of potential issues. Improve your troubleshooting skills with ApnaGuru's comprehensive courses.
For example, if your JavaScript application fails to load, troubleshooting might involve checking network connectivity, verifying the JavaScript file path, inspecting server logs for errors, or even examining browser console messages for hints. Troubleshooting often requires a combination of systematic investigation, problem-solving, and technical knowledge.
<script src="wrong-path/script.js"></script> <!-- Incorrect file path -->
What is Reverse Engineering?
Reverse engineering, in the context of software, is the process of analyzing a program's functionality and structure to understand how it works. This is often done without access to the original source code. It's a powerful technique for understanding complex systems, but it's crucial to respect intellectual property rights and use it ethically. ApnaGuru offers courses to help you understand the ethical implications of reverse engineering.
Consider a situation where you have a compiled JavaScript program and you need to understand its logic. Reverse engineering might involve using decompilers or disassemblers to get a partial view of the original code, then analyzing the code flow to understand what it does. This is a complex process that requires advanced skills and knowledge.
(function(){
var a = "Hidden message";
console.log(a.split('').reverse().join(''));
})();
Using developer tools, you can inspect and deobfuscate such functions.
Debugging in JavaScript
JavaScript debugging is simplified by the rich tools available in modern browsers and integrated development environments (IDEs).
Using the Browser's Developer Tools
Most modern browsers (Chrome, Firefox, Safari, Edge) include integrated developer tools. These tools provide a range of features for debugging, including breakpoints, stepping, variable inspection, and a console for logging messages and executing code. These tools are essential for any JavaScript developer.
The browser's developer tools allow you to step through your code line by line, examining the values of variables at each stage and identifying the precise point where an error occurs. This step-by-step approach makes identifying and fixing bugs incredibly straightforward, and they’re readily available.
Setting Breakpoints
Breakpoints allow you to pause execution at a specific point in your code, enabling a detailed examination of the program's state at that point. To set a breakpoint, you usually click in the gutter next to the line number in the source code view of the developer tools. Learn more about breakpoints and other debugging techniques from ApnaGuru’s expert instructors.
Breakpoints are incredibly powerful in debugging because they allow you to interrupt the normal flow of the program, inspect variables and their values, and trace execution. The ability to pause the execution allows for detailed inspection and a deeper understanding of the execution flow.
function calculate(a, b) {
debugger; // This acts as a manual breakpoint
return a + b;
}
console.log(calculate(2, 3));
Stepping Through Code
Once a breakpoint is hit, you can use the stepping controls in the developer tools to move through the code step by step. This includes stepping over functions (executing the function without stepping into it), stepping into functions (executing line by line within the function), and stepping out of functions (returning to the line after the function call). This allows for a very controlled analysis of the code’s execution.
Stepping through code is critical for understanding the program's state at any point. You can track the flow and how the values of variables change over time, which is invaluable for understanding where a bug might have arisen.
function process() {
let x = 5;
let y = 10;
return x * y;
}
console.log(process());
Inspecting Variables
The developer tools usually include a variable inspector that shows the current values of variables in the current scope. This allows for quick inspection and validation of variable values at any point during execution. This is crucial for understanding if the values are correct at various stages of the program.
let name = "John Doe";
console.log(name); // Inspecting variable
Inspecting variables helps detect inconsistencies and errors in data manipulation. By visually examining the variable values you can compare them against their expected values and quickly pinpoint discrepancies. This quick identification is very valuable in debugging.
Using the Console
The browser console is a powerful tool for logging messages, executing code, and inspecting objects. You can use the console to print variable values, test expressions, and even execute arbitrary code while debugging. This offers flexibility and the ability to test your assumptions.
The console allows you to add statements to output intermediate results during code execution. This gives insight into the code’s progress and helps verify the correctness of various algorithms and data transformations. The console messages are incredibly useful for debugging.
Debugging with IDEs
Integrated Development Environments (IDEs) like Visual Studio Code, WebStorm, and Atom offer advanced debugging features that often surpass those of browser developer tools. These IDEs typically integrate directly with debuggers, providing similar capabilities with added features.
IDEs often provide a more streamlined debugging experience with features such as breakpoints, watch expressions, call stack visualization, and advanced debugging features. These tools greatly enhance the debugging process, making it quicker and easier to find the root cause of errors. The combination of the IDE and debugging tools significantly improves productivity.
Troubleshooting JavaScript Issues
Let's explore the practical aspects of troubleshooting common JavaScript problems.
Common JavaScript Errors
JavaScript errors are often categorized as syntax errors (errors in the structure of the code), runtime errors (errors that occur during code execution), and logical errors (errors in the program's logic that cause incorrect results). Understanding these categories helps in quickly diagnosing problems. ApnaGuru's courses cover a wide range of common JavaScript errors and how to resolve them.
Syntax errors are usually caught by the JavaScript interpreter before execution, while runtime errors and logical errors might only be evident during runtime. Careful analysis of error messages is crucial. Common runtime errors include 'ReferenceError' (accessing an undefined variable), 'TypeError' (performing an invalid operation on a data type), and 'RangeError' (using an index that is out of bounds).
console.log(undefinedVar); // ReferenceError: undefinedVar is not defined
Identifying and Resolving Errors
Effective error identification involves careful examination of error messages, logs, and the code itself. Use of debugging tools like browser developer tools and IDEs is essential. Start by understanding the nature of the error and then systematically investigate the possible causes.
When you encounter errors, look carefully at the error message provided by the JavaScript interpreter. The error message often contains important clues, such as the line number of the error, the type of error, and a short description. Use the error message to pinpoint the section of code that caused the problem.
Using Error Messages
Error messages are your first line of defense against JavaScript issues. Carefully reading and understanding error messages, including line numbers and error types, is crucial. Error messages provide very important clues about the problem.
For example, a 'TypeError: Cannot read properties of undefined (reading 'length')' message clearly points to attempting to access a property of an undefined variable. This helps in narrowing down the scope of the issue and makes debugging less time-consuming.
Debugging Techniques
Employ a systematic approach to debugging. Start by isolating the problematic section of the code and then using debugging tools to step through the execution, inspecting variables and identifying the point of failure. Use logging and console statements liberally to trace data flow.
Techniques like using `console.log()` to print variable values at strategic points in your code are incredibly useful. This provides checkpoints and intermediate results, allowing you to track how data is processed and identify where the program deviates from its intended behavior. The use of print statements is incredibly useful.
console.log("Checking values:", x, y);
Examples of Troubleshooting
Consider a scenario where a JavaScript function designed to calculate the sum of an array is returning incorrect results. Debugging steps would include:
- Setting breakpoints within the function.
- Stepping through the code line by line.
- Inspecting the array contents and intermediate sum values.
- Checking for off-by-one errors in loop indices.
- Verifying the correctness of the summation logic.
This structured approach guides the developer through a thorough analysis and helps in efficiently identifying and resolving the error.
function sumArray(arr) {
let sum = 0;
for (let i = 0; i <= arr.length; i++) { // Off-by-one error
sum += arr[i];
}
return sum;
}
console.log(sumArray([1, 2, 3])); // Error due to incorrect loop condition
Reverse Engineering JavaScript Code
Reverse engineering JavaScript code is a more advanced topic that involves analyzing compiled or obfuscated JavaScript code to understand its functionality. This often requires specialized tools and techniques.
var encrypted = "aGVsbG8gd29ybGQ=";
console.log(atob(encrypted)); // Decodes base64 string
Understanding the Code
Before attempting to reverse engineer JavaScript code, it’s crucial to have a solid understanding of JavaScript concepts, including its syntax, data types, and common programming patterns. The more familiar you are with JavaScript, the easier it will be to interpret the decompiled code.
Understanding the code flow is vital, especially with complex programs. You need to be able to trace the execution through various functions and data structures, observing how data transformations occur. This process often involves careful analysis of the code's control structures, data structures, and algorithms.
Using Decompilers
Decompilers are tools that attempt to reconstruct source code from compiled or obfuscated code. While they cannot perfectly reconstruct the original code, they can provide a valuable starting point for analysis. Many decompilers are available online.
Decompilers are frequently employed for understanding the functionality of JavaScript code that has been minified or obfuscated. Decompiling often produces code that is less readable than the original; however, it provides a framework for understanding the functionality. The decompiled code usually requires significant manual cleanup and interpretation.
Analyzing the Code Flow
Once you have some decompiled or partially deobfuscated code, you need to carefully analyze the code flow. This involves tracing the execution paths through functions and data structures to understand the overall program logic. This often requires debugging tools or other specialized tools.
Flow analysis is often performed visually by creating diagrams or flowcharts to illustrate the various paths of execution. These diagrams allow for a clear representation of the relationships between different parts of the code. This structured approach greatly aids in understanding complex programs.
Modifying the Code
In some cases, you might need to modify the reverse-engineered code. This could involve fixing bugs, adding new features, or adapting the code to a different environment. However, modifying reverse-engineered code can be risky and requires extreme caution.
Modifying reverse-engineered code is a delicate process that requires a deep understanding of the code's functionality. Any changes should be carefully tested and validated to avoid unintended side effects. Changes made without proper understanding could lead to unpredictable behavior.
Ethical Considerations
Reverse engineering should always be conducted ethically and legally. Respect intellectual property rights and avoid reverse engineering code without proper authorization. Always check the license of any software before attempting to reverse engineer it.
Ethical considerations are paramount in software reverse engineering. It is crucial to operate within the bounds of the law and respect the intellectual property rights of others. This is particularly important if the software is proprietary or licensed under a restrictive license.
Advanced Techniques
More sophisticated techniques are also available for debugging, troubleshooting, and reverse engineering JavaScript.
Using Proxies and Logging
Proxies and logging provide powerful tools for monitoring and intercepting function calls and data transformations. Proxies can intercept calls to objects and allow you to log or modify the behavior of the functions. Logging provides a record of the program's execution.
Proxies allow developers to intercept and modify function calls, and logging allows them to record data flow and other events. This provides deeper insight into the code’s operation and aids in identifying potential issues. These techniques are particularly useful for debugging asynchronous code and complex data transformations.
Dynamic Code Analysis
Dynamic code analysis involves analyzing a running program to gather information about its behavior. This can be done using tools that monitor program execution, memory usage, and other aspects. This method is incredibly useful for finding runtime errors or performance issues.
Dynamic analysis typically involves running the program under a controlled environment and monitoring its behavior. The analysis reveals various characteristics including memory usage, execution flow, and the values of variables over time. This offers a broader understanding of the program's operational dynamics.
Static Code Analysis
Static code analysis involves analyzing the code without running it. This can be done using tools that check for coding style violations, potential errors, and security vulnerabilities. Static analysis can find many issues before the code is ever executed, saving time and resources.
Static analysis is usually done by automated tools that scan the code and identify potential problems. The tools check for stylistic inconsistencies and violations of coding standards. The use of automated tools helps find potential issues early in the development cycle, allowing for proactive mitigation of errors.
Code Optimization
Code optimization involves improving the efficiency of a program. This can involve reducing execution time, memory usage, or other performance metrics. Optimization can improve the speed and responsiveness of the program.
Code optimization can be conducted at various stages during development and maintenance. This process requires careful understanding of the code's behavior and the identification of bottlenecks. Techniques like profiling and performance testing are frequently employed to find areas for improvement.
Conclusion
Mastering JavaScript debugging, troubleshooting, and reverse engineering is a continuous process of learning and refinement. The techniques and tools discussed in this blog provide a powerful arsenal for tackling coding challenges. Consistent practice and a systematic approach are key to success.
Remember, effective debugging involves patience, persistence, and the willingness to explore various tools and techniques. By consistently applying the approaches highlighted in this guide, any JavaScript developer can confidently address errors, enhance code quality, and unravel the complexities of JavaScript code.